14 Best Practices For Secure Web Application Development
Web applications often serve as repositories for valuable information, including personal and financial data, as well as login credentials. Hence, implementing secure coding practices for strong security is essential in safeguarding these platforms from hackers and other malicious attacks.
Hence, it is important to practise the necessary strategies to protect web applications against potential breaches and vulnerabilities.
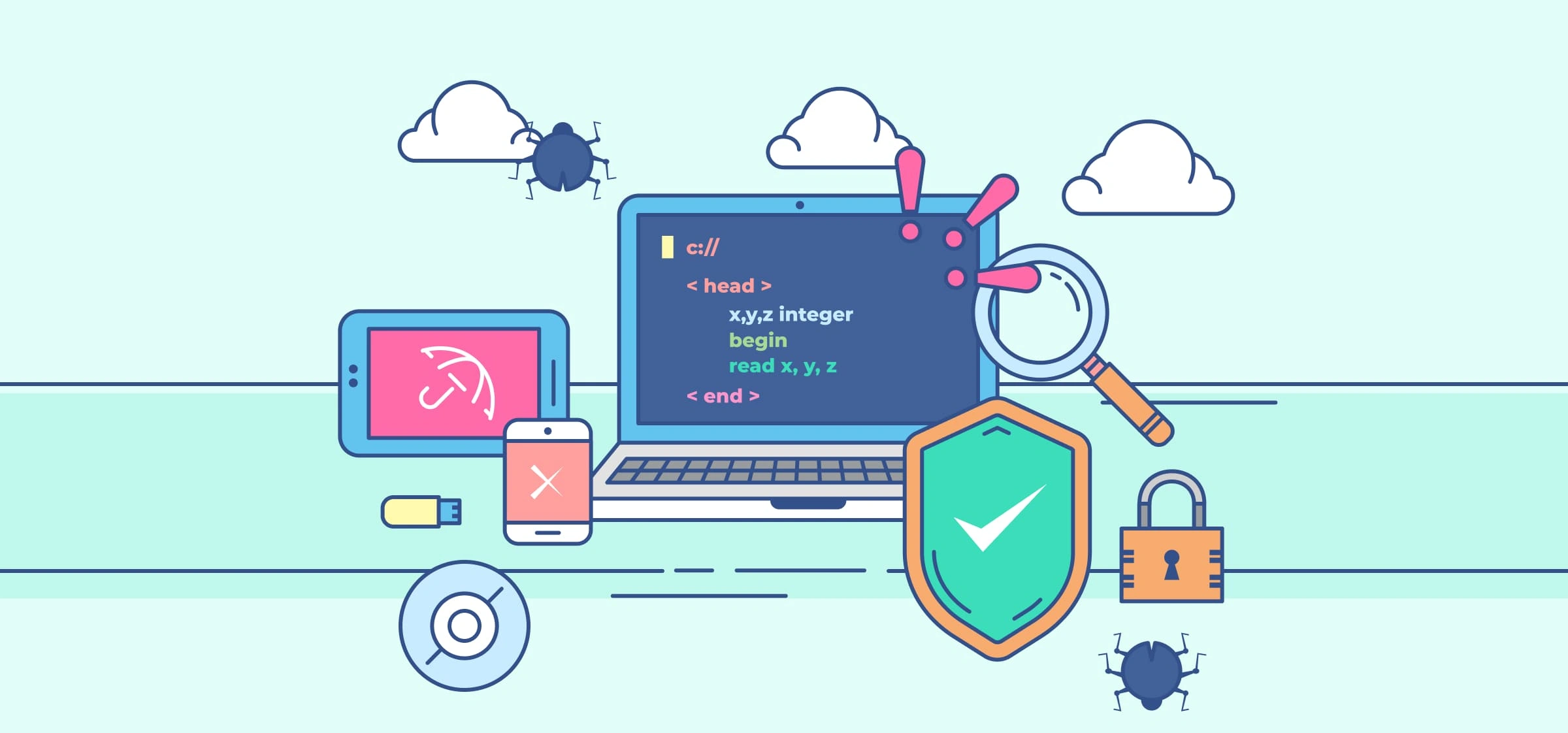
Essential Secure Coding Practices
In this article, we will talk about the 14 best secure coding practices in web application development.
Security Standards Implementation
It is crucial to implement secure coding practices that adhere to established guidelines such as the OWASP Top 10 and CWE Top 25.
These standards provide a framework for identifying and addressing the most critical security risks to web applications.
This proactive approach helps detect and mitigate security issues early in the development cycle, reducing the risk of exploitable vulnerabilities in the final product.
Input Validation
Developers need to validate all the user input to secure web applications during the developmental process in order to protect against attacks such as SQL injections, Cross-Site Scripting (XSS), and command injections.
This process involves checking every piece of user input before processing to ensure the web application adheres to the expected formats, type, and length and contains no harmful content.
Here are some best practices that you follow during the input validation process:
- Define allowable inputs clearly by validating type, format, and length
- Use “positive” or “whitelist” validation to ensure only approved data passes through
- Implement client-side and server-side validation for multiple layers of security
- Leverage frameworks and libraries to avoid SQL injection and other common vulnerabilities
- Regularly update validation logic to respond to new threats
- Test validation mechanisms to ensure effectiveness in blocking harmful data
These secure coding practices can help prevent malicious data from entering the system, thus safeguarding the web application.
Output Escaping
Safeguarding against malicious output can neutralise potential executable content in data being output to users or other systems.
This can prevent attacks like HTML injection. In order to safeguard web applications, developers should practice output encoding.
Here’s how you can practice output escaping:
HTML Escaping
<
, >
, &
, "
, and '
must be converted into their corresponding HTML entities.
- <?php// Original data that might come from user input
- $input = '<script>alert("XSS")</script>';
- // HTML escaping using htmlspecialchars function
- $escapedInput = htmlspecialchars($input, ENT_QUOTES, 'UTF-8');
- echo $escapedInput; // Output: <script>alert("XSS")</script>?>
htmlspecialchars
is used to convert special characters into HTML entities, preventing the execution of malicious scripts. JavaScript Escaping
- <?php// Original data that might come from user input
- $input = 'He said, "Hello, World!"';
- // JavaScript escaping
- $escapedInput = json_encode($input);
- echo '<script>';
- echo 'var userMessage = ' . $escapedInput . ';';
- echo 'console.log(userMessage);'; // Output: He said, "Hello, World!"echo '</script>';
- ?>
json_encode
is used to properly escape the string for safe insertion into JavaScript code. URL Encoding
- <?php// Original data that might come from user input
- $input = 'Hello World! This is a test.';
- // URL encoding
- $encodedInput = urlencode($input);
- echo '<a href="http://example.com/search?q=' . $encodedInput . '">Search</a>';
- // Output: <a href="http://example.com/search?q=Hello+World%21+This+is+a+test.">Search</a>?>
urlencode
is used to convert special characters into their URL-encoded equivalents, ensuring the integrity of the URL. Neutralising Harmful Effects
When it comes to secure coding practices, escaping data appropriately based on the context (HTML, JavaScript, or URL) is a must.
This will neutralize the harmful effects that malicious data could have when executed as code in browsers or other systems.
Using these practices helps protect web applications from various types of injection attacks, including cross-site scripting (XSS) and URL manipulation attacks.
Safe APIs
Insecure APIs can be exploited to alter application behaviour that may lead to unauthorised actions, data manipulation, or service disruptions.
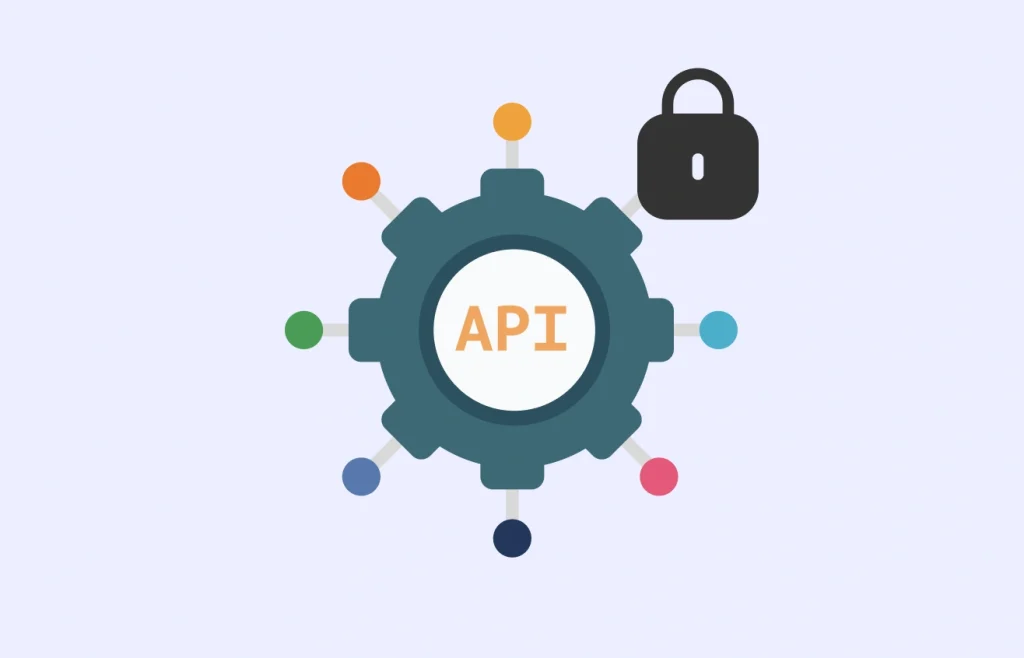
Developers can follow to ensure safe and reliable web application operation:
- Encrypt API data transfers with HTTPS to prevent interception and unauthorized access. This can prevent Man-In-The-Middle (MITM) attacks.
- Implement strong authentication and authorisation mechanisms to control access to the API. Methods such as 0Auth, JWT (JSON Web Tokens), and API keys are commonly used.
- Ensure that all data sent to an API is properly validated to avoid injection attacks and other vulnerabilities.
- Protect APIs from abuse and DoS attacks by limiting how often a user or service can make requests.
Continuously monitor API traffic and maintain logs to promptly detect and respond to suspicious activities.
Web Application Firewall (WAF)
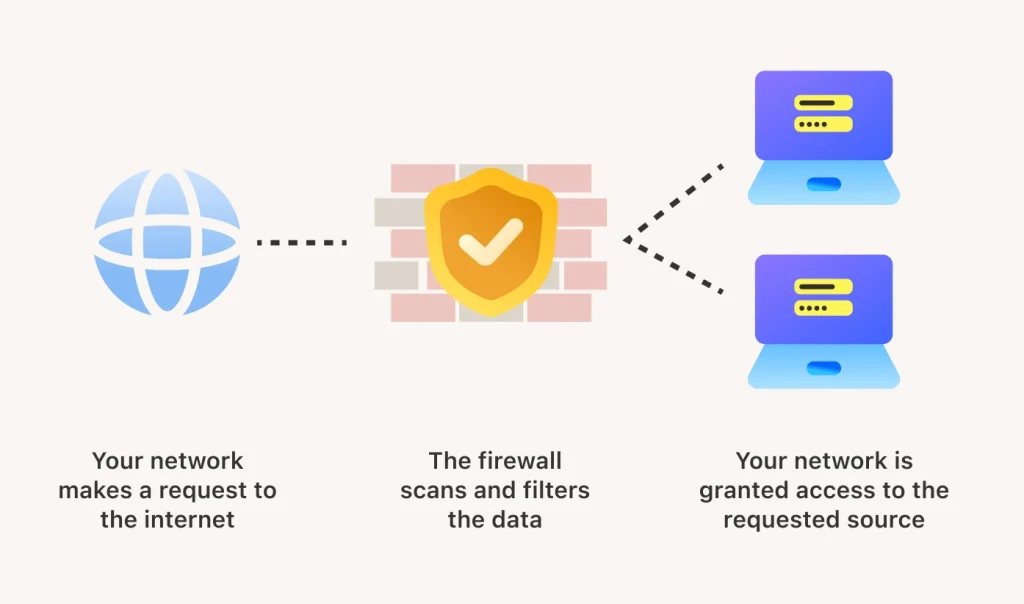
Web Application Firewall, commonly known as WAF, protects web applications from a variety of attacks such as SQL, XSS and other common web-based attacks.
WAF evaluates the incoming HTTP requests and proceeds to block malicious attacks and harmful sites.
Apart from that, it also monitors and filters HTTP traffic between a web application and its users to ensure only safe interactions, ensuring that malicious requests are blocked before they reach the application.
WAF has customisable security rules to fit particular security requirements of your application to best defend against threats that are most likely to target the application.
It indirectly reduces downtime by preventing successful attacks and mitigating threats that could potentially disrupt the application.
In simple terms, it acts as the first-line of defence for the web application.
DevSecOps Adoption
Implementation of DevSecOps (Development, Security, and Operations) aims to build security into web applications from the beginning, rather than adding it later as an afterthought.
Tools such as Static Application Security Testing (SAST), Dynamic Application Security Testing (DAST), Infrastructure as Code (IaC) Security Tools, and vulnerability management tools can be used to create preventive measures against injections attacks, cross-site scripting and sensitive data exposure.
DevSecOps does not only speed up the developmental process but also ensures a higher degree of security throughout the development cycle.
SSL/TLS Usage
Secure Socket Layer (SSL) and Transport Layer Security (TLS), which is the upgraded version of SSL that fixes SSL vulnerabilities, are essential in securing web applications that establish an encrypted link between web server and a browser.
A connection will be established through “SSL/TLS Handshake”, where the browser and server will exchange information to establish a secure encrypted connection. This process will agree on encryption methods and exchange keys for secure communication.
The use of SSL/TLS certificates also provides a level of authentication. These certificates assure users that they are communicating with a legitimate server, not an impostor. The authentication also ensures attackers cannot intercept and read sensitive data.
Data Encryption
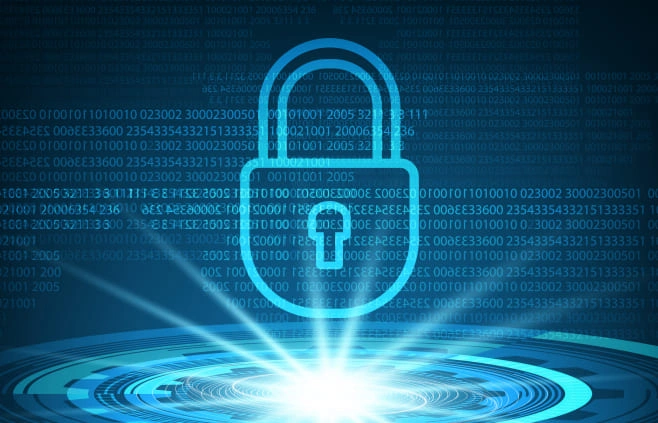
Data encryption will hide the data from those who are not authorised for access although it does not prevent interference in data transmission, making it one of the secure coding practices.
For data in transit encryption, it is best to always use HTTPS instead of HTTP to protect the users’ data.
For instance, when data such as login credentials, financial payments, or personally sensitive information is sent, encryption in transit ensures that it cannot be intercepted and read by unauthorized individuals, especially during Man-In-The-Middle (MITM) attack.
When you visit a website that uses HTTPS, the browser and the server will establish a secure connection, where the server will send its public key and certificate to the browser for decryption.
Meanwhile, encryption at rest is applied to data stored on a server. If someone who’s not authorised gains physical storage (such as a hard drive), they still won’t be able to read the data without encryption keys.
Pen Test
Pen tests are crucial in detecting the logic flaws within the web application that may lead to security breaches as they are often harder to detect.
It will always be one of the secure coding practices that requires developers’ insights to identify scenarios where attackers could exploit flow of the application.
Manual pen tests are able to detect more complex security issues that automated pen test tools might miss.
It helps developers to understand how new features and modifications can cause potential new threats to the web application, especially after having any updates or changes made.
Least Privilege Principle Application
The principle of least privilege only grants users or software components the minimum level of access necessary to perform their tasks or functions.
This principle ensures accounts, programmes and processes operate using the least amount of privilege necessary by restricting access control.
This can limit the access to sensitive parts of the system that are irrelevant to their specific functions.
If an attacker does compromise a user’s account or a component of the application, the attacker can only access the data and functions available to that compromised account or component. With this, the damage they can do is limited to only what they have access to, thus reducing the scope of an attack.
Multi-Factor Authentication (MFA)
-
Something you know: This could be a password or a personal identification number (PIN)
-
Something you have: This could be a mobile phone that can receive a code via text message, or a hardware token
-
Something you are: This involves biometrics, such as fingerprints, facial recognition, or even voice patterns
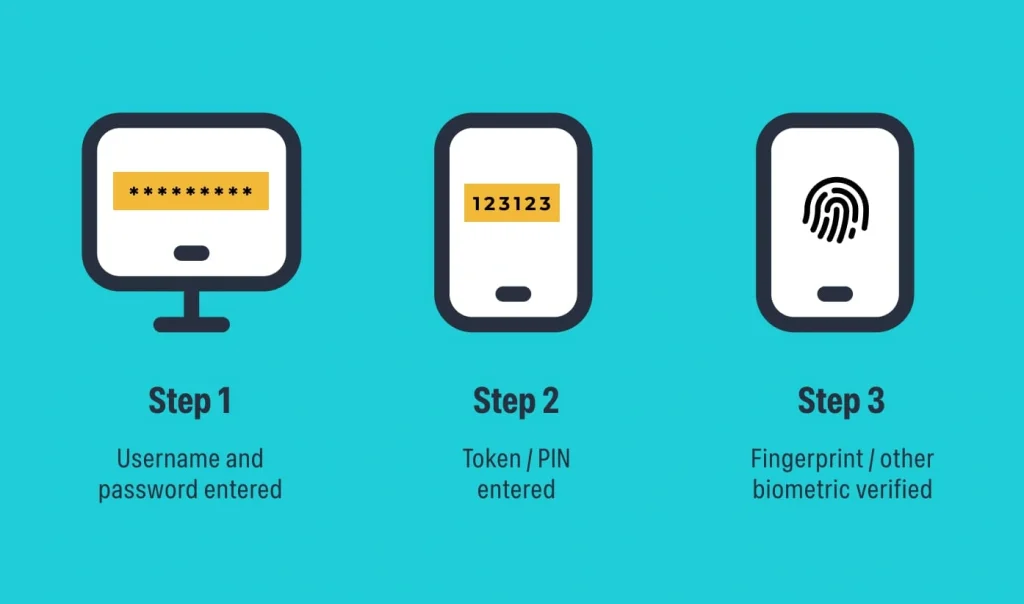
There are many benefits of multi-factor authentication (MFA). As one of the best secure coding practices in web applications, it creates a multi-layered defence that makes it much harder for potential intruders to access devices, networks, or databases.
If one factor is compromised or broken, the attacker still has at least one more barrier to breach before successfully accessing the target.
For example, with MFA, certain apps require you to put a code sent to your email or phone number before logging into the account despite logging in with your password. This acts as evidence that is used to confirm your identity.
Hence, the breach of one authentication factor alone becomes insufficient for the attacker.
Secure Session Management
A session in web application terms is a way of maintaining a conversation between the user and the application, where the session remembers the user’s login details and browser activity from the time they login to the time they log out.
When there’s inactive activity, sessions will automatically expire after a certain period of time or after the purpose of the session is fulfilled, preventing unauthorised access.
For instance, banking apps often log out automatically after being inactive for a certain duration of time. This is because each session has an identifier that will be transmitted securely using encryption like HTTPS to prevent interception by eavesdroppers.
Marking cookies as HttpOnly
ensures they cannot be accessed via JavaScript, mitigating the risk of cross-site scripting (XSS) attacks.
Marking cookies as Secure
ensures they are only sent over HTTPS connections, protecting them from being intercepted by attackers during transmission such as man-in-the-middle attack.
Regular Updates & Patches
Software that isn’t regularly updated or patched after its initial release can become vulnerable to security threats or fail to function correctly as surrounding technologies evolve.
This is because hackers continually find and exploit vulnerabilities, including zero-day vulnerabilities—previously unknown flaws that attackers can exploit before developers have a chance to address them.
Patches often fix bugs that can affect the performance of software and resolve issues that could cause the software to crash.
Regularly applying patches specifically made for bugs or performance issues can fix these vulnerabilities and improve the overall stability and security of the software.
Error Handling & Logging
Error handling and logging is one of the secure coding practices that should not be left out to achieve a robust web application. Here’s how it helps to safeguard a web app:
Error Handling
Error handling is a programming practice used to anticipate, detect, and resolve problems that may occur during the execution of an application.
Error handling prevents application crashes by catching errors and dealing with them in a way that doesn’t negatively affect the user experience or security.
Users will receive clear and helpful messages when something goes wrong, without exposing sensitive information like system details or underlying security mechanisms.
Through this, the application can recover from an error, allowing the user to continue using the application whenever possible.
In a production environment, it is crucial to catch errors without revealing sensitive data or code details to the users. This maintains the security and integrity of the application. However, in a development environment, displaying detailed error messages is beneficial as it helps developers debug and resolve issues more efficiently.
Logging
Logging involves recording events that occur while software is running. It carries info such as data about application operations, system events, and user actions.
Logs provide a historical record of events to developers and system administrators to help diagnose and fix issues.
With this, developers can detect any security incidents such as unauthorised access attempts or suspicious activities to ensure that the security controls are functioning correctly.
They can also identify the components that are performing poorly and thus proceed to address the issue by providing updates and patches.
Conclusion
By integrating these security measures into the development process, developers can build and maintain applications that can stand strong against cyber threats.
Implementing these secure coding practices can ensure the web applications stay powerful, efficient and shielded to the core!