Avoid These Front-End Development Mistakes!
Avoiding common front-end development mistakes is essential if you want your website or web app to perform optimally and provide a smoother user experience.
You can keep your code clean, efficient, and user-friendly with a few simple tips.
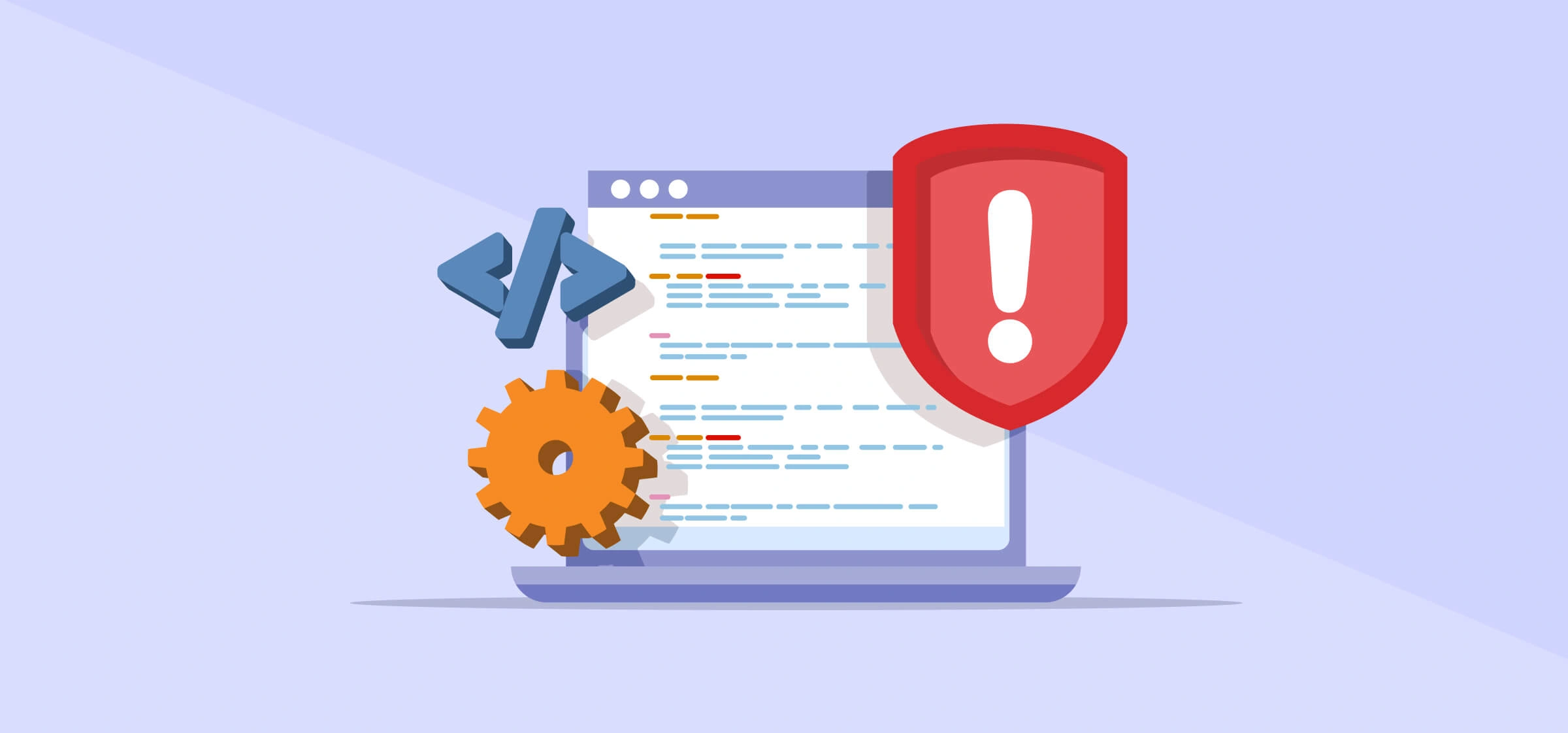
Using Inline Styles
Inline styles can seem quick and much easier to apply specific styles to an element. However, if you want to change colour or button size, you will have to edit every single element with a <style>
attribute.
Besides, inline styles in HTML may not be the best for browsers as they aren’t cacheable and may not render the styles efficiently.
Hence, it is best to avoid inline styles not just because most developers said so, but because even Google recommends keeping the interaction between styling, markup and scripting minimal.
Solution:
Use external CSS files: Separate your styles into dedicated .css files. This improves maintainability, allows for better organization, and enables browser caching for improved performance.
HTML
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>External Styles Example</title> <link rel="stylesheet" href="styles.css"> </head> <body> <h1>This is a Heading</h1> <p>This is a paragraph styled with an external stylesheet.</p> </body> </html>
style.css
h1 { color: blue; font-size: 24px; } p { color: green; font-size: 16px; }
Ignoring Cross-Browser Compatibility
Better Approaches:
1. Prioritise testing on popular browsers. Focus on recent versions of Chrome, Firefox, Safari, and Edge.
2. Use tools like Normalize.css to help establish a consistent baseline across browsers.
a. Example
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Normalize.css Example</title> <!-- Include Normalize.css from a CDN --> <link rel="stylesheet" href=" https://cdnjs.cloudflare.com/ajax/libs/normalize/8.0.1/normalize.min.css "> <!-- Your custom styles --> <style> /* Your styles here will build upon the normalized baseline */ body { font-family: Arial, sans-serif; line-height: 1.6; padding: 20px; } h1 { color: #333; } </style> </head> <body> <h1>Welcome to my normalized page</h1> <p>This page uses Normalize.css to ensure a consistent baseline across different browsers.</p> </body> </html>
3. Start with a basic version that works everywhere, then add advanced moden features for modern browsers.
4. Utilise cross-browser testing tools to test on various browser/device combinations.
5. Use autoprefixers to automatically add vendor prefixers to CSS properties for better compatibility.
a. Before autoprefixer
.example { display: flex; transition: transform 1s; user-select: none; background-image: linear-gradient(to bottom, #fff, #000); }
b. After autoprefixer
.example { display: -webkit-box; display: -ms-flexbox; display: flex; -webkit-transition: -webkit-transform 1s; transition: -webkit-transform 1s; transition: transform 1s; transition: transform 1s, -webkit-transform 1s; -webkit-user-select: none; -moz-user-select: none; -ms-user-select: none; user-select: none; background-image: -webkit-gradient(linear, left top, left bottom, from(#fff), to(#000)); background-image: linear-gradient(to bottom, #fff, #000); }
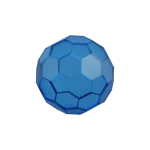
Learn how to fix cross-browser compatibility issues when developing websites and web applications.
Neglecting Image & Video Optimisation
Many front-end developers underestimate the impact of unoptimized media files on website performance.
Large image and video files can significantly slow down your website, leading to poor user experience, increased bounce rates, and potentially negative impacts on search engine rankings.
Solution:
1. Optimise images:
- Convert images to modern formats like WebP, which offer better compression without quality loss.
- Use tools like ImageOptim, TinyPNG, or Squoosh for efficient image compression.
- Implement responsive images using the
srcset
attribute to serve appropriate sizes for different devices.
2. Implement lazy loading
- Use the
loading="lazy"
attribute on images and iframes not immediately visible on page load. - For older browsers, consider using Intersection Observer API or lazy loading libraries.
<picture> <source srcset="image.webp" type="image/webp"> <source srcset="image.jpg" type="image/jpeg"> <img src="image.jpg" alt="Description" loading="lazy"> </picture>
3. Handle videos carefully
- Compress videos without significant quality loss using tools like HandBrake.
- For larger files, consider hosting videos on platforms like YouTube or Vimeo and embedding them on your site.
- Use adaptive bitrate streaming for longer videos to adjust quality based on the user’s connection speed.
4. Leverage browser caching
- Set appropriate cache headers for your media files to reduce server requests on repeat visits.
Inconsistent Code Formatting
<html> <head> <title>Inconsistent Code</title> <style> h1{font-size:22px; color:green} p {font-size:14px;color:red;} </style> </head> <body> <h1>Inconsistent heading</h1> <p>Text with inconsistent code</p> <script> function showAlert(){alert('Hello!');} var variable = 5; if(variable>2){alert('Variable is greater than 2');} </script></body> </html>
Solution:
1. Set clear coding guidelines:
Use a separate file for HTML, CSS and JavaScript for a clear structure when there is more than one developer contributing to the same project.
Utilise linters to catch errors and inconsistencies and formatters to ensure everyone in your team writes the code in the same style.
HTML:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Consistent Code</title> <link rel="stylesheet" href="style.css"> </head> <body> <h1>Consistent Heading</h1> <p>Text with consistent code</p> <script src="script.js"></script> </body> </html>
style.css:
h1 { font-size: 22px; color: green; } p { font-size: 14px; color: red; }
script.js:
function showAlert() { alert('Hello!'); } const variable = 5; if (variable > 2) { alert('Variable is greater than 2'); }
2. Set up pre-commit hooks:
Use tools like Husky to run linters and formatters before each commit. This will ensure code styles across the team is consistent.
For instance, you can include the following Husky configuration in your package.json
file.
{ "scripts": { "lint": "eslint .", "lint:fix": "eslint . --fix", "format": "prettier --write ." }, "husky": { "hooks": { "pre-commit": "npm run lint && npm run format" } } }
You will need to install Husky, ESLint, and Prettier in your project: npm install --save-dev husky eslint prettier
.
This will help to automatically run the linter and formatter before each of your commit, ensuring that your codes will always be formatted.
3. Use consistent naming conventions:
Adopt clear and consistent naming for variables, functions, classes, and file names. These naming conventions help maintain consistency across your codebase:
- Variables and funcions, use camelCase.
// Variables let userName = 'John Doe'; const maxLimit = 100; // Function function calculateTotal(price, quantity) { return price * quantity; }
- Classes use PascalCase.
class UserProfile { constructor(name, email) { this.name = name; this.email = email; } }
- Constants use UPPER_SNAKE_CASE.
const MAX_USERS = 100; const API_BASE_URL = 'https://api.example.com';
- File names generally use kebab-case, except for React components which use PascalCase.
// File names: Use kebab-case for most files user-profile.js api-service.js // Component files in React: Use PascalCase UserProfile.jsx NavigationBar.jsx
- CSS classes use kebab-case.
.user-profile { background-color: #f0f0f0; } .user-name { font-weight: bold; }
By following these conventions:
- Your code becomes more readable and predictable.
- It’s easier for team members to understand and navigate the codebase.
- You reduce the cognitive load when switching between different parts of your project.
Ignoring Responsive Design
A non-responsive design will drive away visitors and lead to poor mobile experience. Users often access websites on both mobile and desktop.
Solution:
1. Design with a mobile-first approach in mind.
2. Use flexible layouts (e.g., Flexbox or Grid).
3. Implement responsive images.
4. Utilise CSS media queries to adjust layouts and styles based on screen size.
a. Example on media queries usage:
/* Base styles (mobile-first approach) */ .container { width: 100%; padding: 15px; } .nav-menu { display: none; /* Hide menu by default on small screens */ } /* Tablet and larger screens */ @media screen and (min-width: 768px) { .container { width: 750px; margin: 0 auto; } .nav-menu { display: flex; /* Show menu on larger screens */ justify-content: space-between; } } /* Desktop screens */ @media screen and (min-width: 1024px) { .container { width: 960px; } }
- Extra small (xs): < 576px
- Small (sm): ≥ 576px
- Medium (md): ≥ 768px
- Large (lg): ≥ 992px
- Extra large (xl): ≥ 1200px
- Extra extra large (xxl): ≥ 1400px
These additional breakpoints allow for finer control over how your layout adapts across a wider range of devices.
The exact breakpoints you choose should depend on your specific design and the devices your users are likely to use.
Neglecting Accessibility
Solution:
Add semantic HTML elements such as <header>
, <nav>
, and <footer>
to help search engine crawlbots, screen readers or other user devices to understand the structure and meaning of the content for better navigation and comprehension.
Don’t forget to provide alt text for images and use contrast colours for readability. Ensure your entire website or web app is navigable via keyboard shortcuts.
<!-- 1. Semantic HTML --> <header> <h1>Website Title</h1> <nav> <ul> <li><a href="#home">Home</a></li> <li><a href="#about">About</a></li> <li><a href="#contact">Contact</a></li> </ul> </nav> </header> <main> <article> <h2>Article Title</h2> <p>Article content goes here...</p> </article> </main> <footer> <p>© 2024 Your Company</p> </footer> <!-- 2. Text alternatives --> <img src="logo.png" alt="Company Logo:A blue circle with a white star"> <!-- 3. Keyboard navigation --> <button onclick="submitForm()" onkeypress="submitForm()">Submit</button> <!-- 4. Color contrast (This would be in your CSS) --> <style> body { color: #333; /* Dark gray text */ background-color: #fff; /* White background */ } </style> <!-- 5. Accessible forms --> <form> <label for="name">Name:</label> <input type="text" id="name" name="name" required> <label for="email">Email:</label> <input type="email" id="email" name="email" required> <button type="submit">Submit</button> </form> <!-- 6. ARIA labels for complex widgets --> <div id="tabs" role="tablist"> <button id="tab1" role="tab" aria-selected="true" aria-controls="panel1">Tab 1</button> <button id="tab2" role="tab" aria-selected="false" aria-controls="panel2">Tab 2</button> </div> <div id="panel1" role="tabpanel" aria-labelledby="tab1"> Content for Tab 1 </div> <div id="panel2" role="tabpanel" aria-labelledby="tab2" hidden> Content for Tab 2 </div>
These examples demonstrate key accessibility features:
- Semantic HTML helps screen readers understand the structure of your content.
- Alt text provides context for images when they can’t be seen.
- Adding keyboard events ensures functionality for non-mouse users.
- Proper colour contrast improves readability for all users.
- Labeled form inputs help users understand what information is required.
- ARIA attributes provide additional context for complex interactive elements.
Remember, these are just starting points. The Web Content Accessibility Guidelines (WCAG) provide comprehensive guidance on making web content more accessible.
Tools like the WAVE Web Accessibility Evaluation Tool or the built-in accessibility auditors in browser developer tools can help you identify and fix accessibility issues.
Forgetting SEO Optimization
If you want to target search engine ranking, common front-end mistakes will significantly affect your site’s visibility.
Improper use of header tags, neglecting meta tags and failing to optimise images can negatively impact your ranking.
Solution:
Semantic HTML comes in hand to create clear hierarchical structures with header tags such as <h1>
, <h2>
, etc.
Check with a content logical flow tool. Ensure every page has meta titles, descriptions and alt attributes.
Use proper tags like <header>
, <nav>
, <main>
, <article>
, and <footer>
.
Meta tags:
- Proper
<title>
tag Meta description
<meta name="description" content="A brief, compelling description of your page content (150-160 characters).">
-
Open Graph tags for better social media sharing
<meta property="og:title" content="Your Page Title"> <meta property="og:description" content="A brief description for social media sharing."> <meta property="og:image" content="https://yourdomain.com/path-to-image.jpg"> <meta property="og:url" content="https://yourdomain.com/page-url">
Implement lazy loading for images and compress your CSS and JavaScript files to improve pagespeed. This can help you to optimise your website for SEO and help to drive the organic traffic you need for high search rankings.
Image optimization:
- Using descriptive
alt
text Implementing lazy loading with the
loading="lazy"
attribute
<img src="image.jpg" alt="Descriptive alt text" loading="lazy">
Page speed optimization:
- Linking to minified CSS and JavaScript files.
Using
defer
attribute on script tag to prevent render-blocking.
<script src="script.min.js" defer></script>
Mobile-Friendliness:
- Including the viewport meta tag for responsive design.
<meta name="viewport" content="width=device-width, initial-scale=1.0">
Structured data:
- Implementing JSON-LD for rich snippets in search results
<!-- Structured data for rich snippets --> <script type="application/ld+json"> { "@context": "https://schema.org", "@type": "Article", "headline": "Article Headline", "author": { "@type": "Person", "name": "Author Name" }, "datePublished": "2024-01-15T08:00:00+08:00", "image": "https://yourdomain.com/article-image.jpg" } </script>
Conclusion
Common front-end mistakes are not inevitable with the right strategy. As long as you address the issues, you can save time together with your team while also providing a superior user experience.
Hope this article can help you build a functional website!
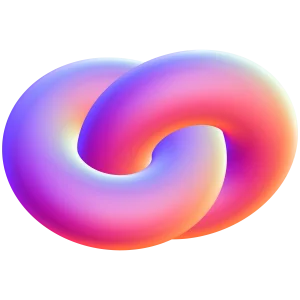
Read the best practices for a secure coding in web application development.