Creating Multi-Language Flutter Apps with Localisation
Building an app that cater to diverse audiences is crucial when a business expands globally. One key way to deploy an app that speaks multi-language is through localisation in Flutter.
In this article, we will guide you through creating a multi-language Flutter app using localisation.
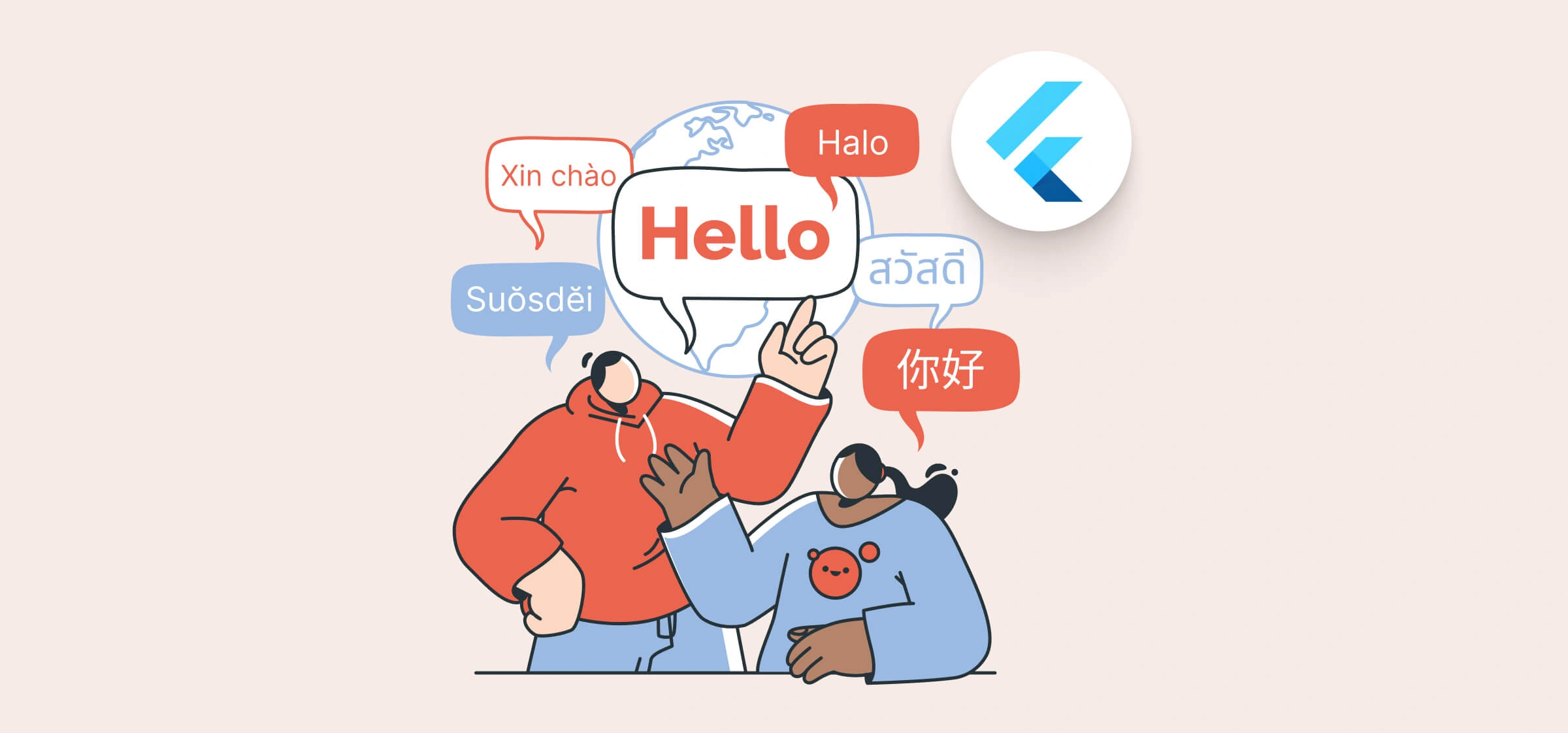
Why You Should Implement Localisation?
Localisation is the process of adapting your app to different languages and cultural norms. This increases user experience and are likely to increase the number of users too.
It covers aspects beyond translation:
- Formatting: Dates, numbers, and currencies vary across regions.
- Right-to-Left (RTL) Support: Languages like Arabic and Hebrew require specific layout adjustments.
- Cultural Sensitivity: Ensuring images, colours, and text resonate with the target audience.
Key Concepts In Flutter Localisation
Internationalisation (i18n)
Preparing your app’s codebase to support multiple languages.
Localisation (i10n)
Adapting the content and layout to specific language and region.
Locales
Identifiers for language and regional settings (e.g., en_my
for English in Malaysia or en_ph
for English in Philippines).
How To Localise A Flutter App?
Step 1: Create A New Flutter Project
Start by creating a new Flutter project. Open your terminal and run:
- flutter create multi_language_app
Step 2: Add Dependencies
To simplify localization, add the flutter_localizations
package and optionally the intl
package for date and number formatting. Modify your pubspec.yaml
file:
- dependencies:
- flutter:
- sdk: flutter
- flutter_localizations:
- sdk: flutter
- intl: ^0.18.0
Run flutter pub get
to fetch the packages.
Step 3: Configure Supported Locales
In your main.dart
, import the necessary localization packages and configure your app to support multiple locales:
- import 'package:flutter/material.dart';
- import 'package:flutter_localizations/flutter_localizations.dart';
- void main() => runApp(MyApp());
- class MyApp extends StatelessWidget {
- @override
- Widget build(BuildContext context) {
- return MaterialApp(
- title: 'Multi-Language App',
- supportedLocales: [
- Locale('en', 'US'),
- Locale('es', 'ES'),
- Locale('fr', 'FR'),
- ],
- localizationsDelegates: [
- GlobalMaterialLocalizations.delegate,
- GlobalWidgetsLocalizations.delegate,
- GlobalCupertinoLocalizations.delegate,
- ],
- home: HomeScreen(),
- );
- }
- }
- class HomeScreen extends StatelessWidget {
- @override
- Widget build(BuildContext context) {
- return Scaffold(
- appBar: AppBar(title: Text('Multi-Language App')),
- body: Center(child: Text('Hello, World!')),
- );
- }
- }
Step 4: Create Translation Files
Use the intl
package to manage translations. Create an l10n
folder in your project and add JSON files for each supported language:
l10n/en.json
:
- {
- "title": "Multi-Language App",
- "greeting": "Hello, World!"
- }
l10n/es.json
- {
- "title": "Aplicación Multi-Idioma",
- "greeting": "¡Hola, Mundo!"
- }
l10n/fr.json
- {
- "title": "Application Multi-Langues",
- "greeting": "Bonjour le Monde!"
- }
Step 5: Create A Localisation Class
Build a localisation class to load and access translations. Create a new file app_localizations.dart
:
- import 'dart:convert';
- import 'package:flutter/material.dart';
- import 'package:flutter/services.dart';
- class AppLocalizations {
- final Locale locale;
- AppLocalizations(this.locale);
- static AppLocalizations? of(BuildContext context) {
- return Localizations.of<AppLocalizations>(context, AppLocalizations);
- }
- late Map<String, String> _localizedStrings;
- Future<void> load() async {
- String jsonString = await rootBundle.loadString('l10n/${locale.languageCode}.json');
- Map<String, dynamic> jsonMap = json.decode(jsonString);
- _localizedStrings = jsonMap.map((key, value) => MapEntry(key, value.toString()));
- }
- String translate(String key) {
- return _localizedStrings[key] ?? key;
- }
- static const LocalizationsDelegate<AppLocalizations> delegate = _AppLocalizationsDelegate();
- }
- class _AppLocalizationsDelegate extends LocalizationsDelegate<AppLocalizations> {
- const _AppLocalizationsDelegate();
- @override
- bool isSupported(Locale locale) => ['en', 'es', 'fr'].contains(locale.languageCode);
- @override
- Future<AppLocalizations> load(Locale locale) async {
- AppLocalizations localizations = AppLocalizations(locale);
- await localizations.load();
- return localizations;
- }
- @override
- bool shouldReload(covariant LocalizationsDelegate<AppLocalizations> old) => false;
- }
Step 6: Update main.dart
Update your main.dart
to use the custom localisation class:
- import 'app_localizations.dart';
- class MyApp extends StatelessWidget {
- @override
- Widget build(BuildContext context) {
- return MaterialApp(
- title: 'Multi-Language App',
- supportedLocales: [
- Locale('en', 'US'),
- Locale('es', 'ES'),
- Locale('fr', 'FR'),
- ],
- localizationsDelegates: [
- AppLocalizations.delegate,
- GlobalMaterialLocalizations.delegate,
- GlobalWidgetsLocalizations.delegate,
- GlobalCupertinoLocalizations.delegate,
- ],
- home: HomeScreen(),
- );
- }
- }
- class HomeScreen extends StatelessWidget {
- @override
- Widget build(BuildContext context) {
- final localizations = AppLocalizations.of(context);
- return Scaffold(
- appBar: AppBar(title: Text(localizations?.translate('title') ?? '')),
- body: Center(child: Text(localizations?.translate('greeting') ?? '')),
- );
- }
- }
This helps ensure that unused Java/Kotlin code doesn’t bloat your final APK.
Step 7: Test Your App
Run your app and switch the device language to test localization. The text should automatically adapt to the selected language.
Additional Tips for Flutter Localisation
1. Use intl
to format dates, currencies, and numbers based on locale.
- import 'package:intl/intl.dart';
- String formattedDate = DateFormat.yMMMd('en_US').format(DateTime.now());
2. Add Directionality
widgets to handle right-to-left layouts.
3. Use tools like Flutter Intl
or third-party services for managing translations.
Conclusion
Creating a multi-language Flutter app is a straightforward process when you understand the localisation workflow. Flutter’s rich localisation features and the step-by-step guide outlined above make the process accessible and efficient.
With these guide, you can confidently create apps that resonate with users worldwide, driving engagement and expanding your market presence.
Don’t forget to read the Flutter documentation if you need more help in implementing localisation.
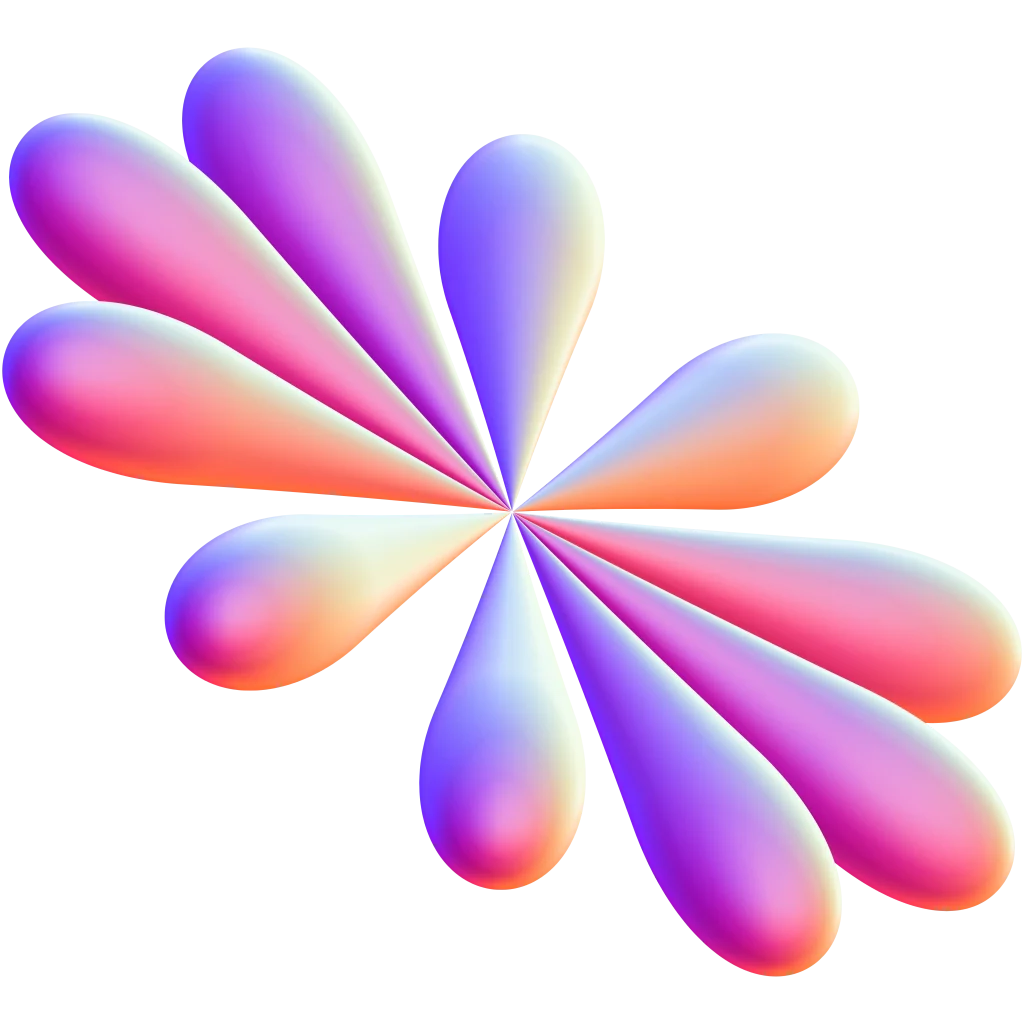
If you are struggling on reducing your Flutter app size, don’t miss this comprehensive guide.
Build An App That Speaks Every Language!
Partner with us to build a multilingual app that fits your project goals!
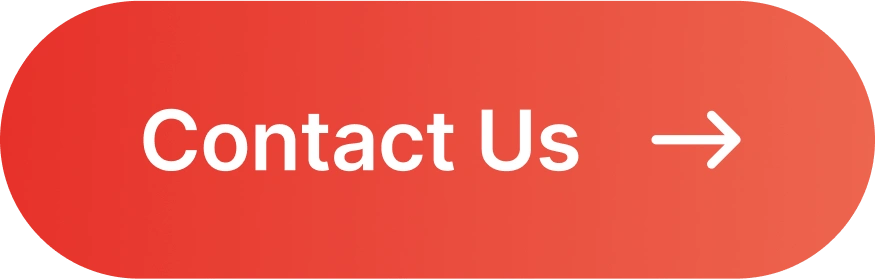