Best Practices For Reducing Flutter App Size
Large app sizes can discourage users from installing and keeping an app, hence why you need to reduce the Flutter app size. Smaller app sizes reduce storage consumption on devices, This can significantly improve user adoption, retention, and overall satisfaction for users with limited storage.
A smaller codebase means cleaner and maintainable code. While reducing the size doesn’t directly equal better run-time performance, it frequently encourages mobile developers to optimise their code structure.
This article will guide you with practical tips, techniques, and insights into how to reduce Flutter app size.
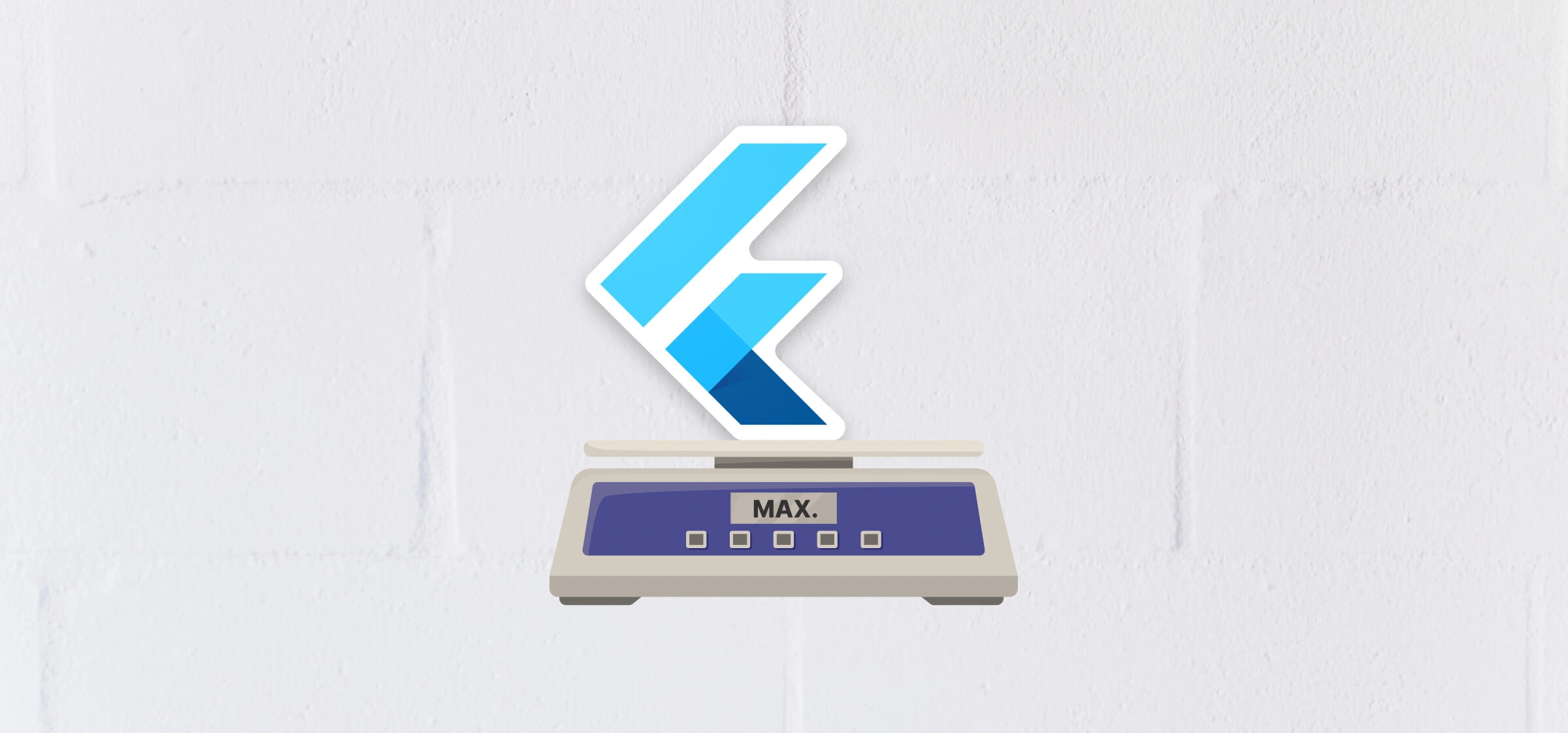
Analyse App Size with Flutter Tools
Before optimizing, analyze your app size to understand the contributors to its bloat. Use the flutter build
command to generate a size analysis report. Running flutter build apk --analyze-size
or flutter build appbundle --analyze-size
can pinpoint which parts of your app consume the most space.
The Flutter DevTools contains a app size
tab that helps visualise the size breakdown. This view provides insights into libraries, packages, and assets that might inflate your app.
Leverage App Bundles or Split APKs
Run flutter build appbundle
for Android. Google Play requires the Android App Bundle (.aab) format, which supports dynamic delivery. This ensures users only download the resources needed for their specific device configurations like CPU architecture and language, resulting in smaller initial downloads. For iOS, ensure Xcode’s Bitcode is enabled for better optimization during app submission.
If you still need APK releases for specific use cases, consider splitting them by Application Binary Interface (ABI) using Gradle. This way, users only download the APK optimized for their device, reducing redundant binaries.
Command Example:
- flutter build apk --split-per-abi
Remove Third-Party Dependencies
Every additional package or plugin potentially adds extra code and resources. This may also cause an increase in app size. Regularly audit your pubspec.yaml
file. Remove packages that are no longer in use or replace them with a lighter solution.
Investigate the dependencies’ impact on the app by running command below to view the transitive packages. Replace heavier dependencies with custom implementations if feasible. Please run this in project’s Command Line Interface (CLI):
- pub deps --style=compact
Encourage your team to adopt a “less is more” approach by questioning whether each dependency adds significant value to the app.
Optimise Image Assets
Use image compression tools or any online services to compress images before bundling them into your app. Consider replacing PNGs and JPGs with SVGs where feasible as they scale better on different screens. For more responsive images, serve different image sizes based on screen density using Flutter’s AssetImage API.
Replace bitmap images with vector assets using Flutter’s flutter_svg
package to achieve smaller file sizes.
Minimise Font Usage
Custom fonts can quickly inflate app size. Reduce their impact by subsetting fonts to include only the characters you need. Try using system fonts whenever possible as they can eliminate the need for embedding font files.
Use Google Fonts’ API to dynamically fetch fonts rather than embedding them directly into your app, saving space for multilingual applications.
Utilise Tree Shaking & Code Shrinking
Enable Tree Shaking to reduce the number of icon bundles. For example, you can use --tree-shake-icons
. Ensure you build your app in release mode (flutter build apk --release
or flutter build ios --release
) to enable tree shaking.
Please run this in project’s Command Line Interface (CLI) to generate the build:
- flutter build apk --release --tree-shake-icons
Dart’s compiler optimizations ensure unused code is stripped out in release builds. Keep your code lean and modular with Dart’s compiler optimisation. This ensures unused code strips out in the release build. Avoid code duplication and keep an eye on large classes that might pull in unnecessary dependencies.
When building a release version of your app, consider using the --split-debug-info tag
to reduce code size.
Use Flutter’s Defer Loading and Code Splitting
Split your codebase by separating large functionalities into separate modules or packages that can be triggered after the initial code. Flutter doesn’t have traditional code splitting, but you can still structure your project to minimal dependencies on critical paths.
For platform-specific code or rarely used features, use conditional imports and load the code only when needed. This prevents all the integrations from being bundled upfront.
Use ProGuard/R8 in Android Builds
- buildTypes {
- release {
- shrinkResources true
- minifyEnabled true
- proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro'
- }
- }
This helps ensure that unused Java/Kotlin code doesn’t bloat your final APK.
Avoid Large Native Libraries
If your app integrates native libraries, use lightweight alternatives or remove libraries with overlapping functionalities. Use only the essential parts of large libraries like Firebase by modularizing them in your pubspec.yaml
file (e.g., firebase_core
, firebase_auth
, etc.).
Keep Flutter and Dart SDKs Updated
Use the latest stable SDK. Newer versions of Flutter and Dart are often better optimized in terms of build sizes and performance. Keep an eye on Flutter’s release notes and upgrade when stable releases are available.
Monitor Continuously
Implement automated size checks in your continuous integration workflow. Monitor the changes in app size over time to catch any regressions and maintain a lean application.
Another method you can implement is to set size thresholds for your app and use them as benchmarks. If an incremental feature pushes you over your target size, consider optimisations before release.
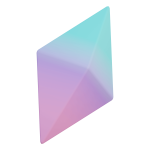
Learn how to integrate CI/CD workflow tools with Flutter.
Educate Your Stakeholders
Lastly, reducing app size is not just a developer’s concern. Project managers, business owners, and other stakeholders should understand that smaller app sizes can lead to higher install rates, better user engagement, and potentially higher revenue.
During the product planning phase, consider the trade-offs between feature complexity and app size. Encourage a culture of optimization across the entire product lifecycle.
Advocate for lightweight MVPs during initial development phases to build a solid foundation that scales without excessive bloat.
Conclusion
Optimising your Flutter app to reduce the size is a technical necessity and a business advantage. Smaller apps lead to higher user retention, improved download rates, and better user experience. By following these strategies, you can ensure your app remains competitive and appealing in the market.
Refer to the official Flutter app size guide and related community resources for additional insights and tools.
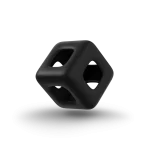
Explore Flutter state management libraries insights from our developer.
Build your Flutter project with us.
Make your app smaller, faster, and more user-friendly today!
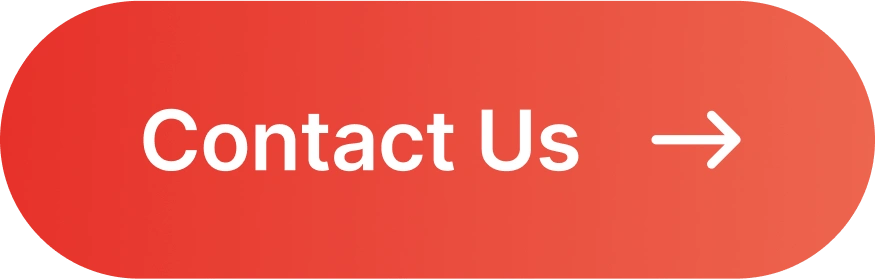